I’m working on a custom integration for our app, where we would want to query klaviyo to get a profile ID from the cookie or session somehow. Meaning, what I’m trying to do is be able to retrieve a profile ID from the cookie or something so i can map it back to a call for the full profile etc.
Is this somehow possible? Can’t seem to find the api docs or guide on how we would be able to identify a klaviyo profile/tracked user on the merchant site while the site is loading/page loaded etc.
The __kla_id cookie is a base64-encoded JSON block. If there’s an identified profile, there will be a property such as:
"$exchange_id":"bIgfUuOCPJUzy9lKtTkDmnAS8EE6u0FxfWDk07E-sAytXAgIwM3cG9F216V5Gvmn.PGuJQv"
You can use this value in a filter in Get Profiles in a server-side call:
https://developers.klaviyo.com/en/reference/get_profiles
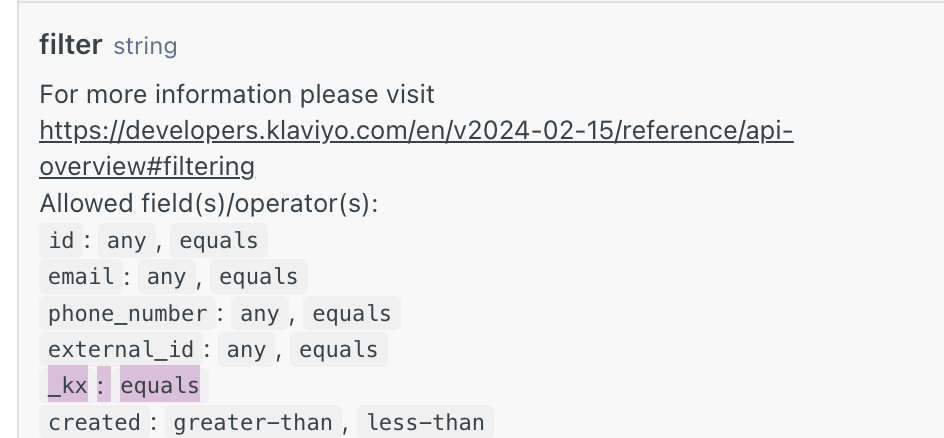
This would give you the profile ID.
No PII is exposed in the cookie itself, so you’d have to make this server-side API call.
Is possible to display the username a webpage with _kla_id?
Is possible to display the username a webpage with _kla_id?
The __kla_id cookie does not store any profile PII. See the earlier answer to make a server-side call to Get Profile to fetch the Klaviyo profile, and its properties like email, and first and last name.
Is possible to display the username a webpage with _kla_id?
The __kla_id cookie does not store any profile PII. See the earlier answer to make a server-side call to Get Profile to fetch the Klaviyo profile, and its properties like email, and first and last name.
So is the correct method
Step 1: Extract __kla_id
Cookie
Use JavaScript to retrieve the __kla_id
cookie and decode it to obtain the _kx value.
Step 2: Exchange _kx for Profile ID
Make a POST request to Klaviyo’s /api/v2/people/exchange
endpoint with the _kx value to retrieve the profile ID.
Step 3: Get Profile Details
Use the GET /api/profiles
endpoint with the profile ID to fetch the profile data, including the first name.
Step 4: Display First Name
Once you get the first name from the API, update the HTML element with the user's name.
The v2 APIs are deprecated; use Get Profiles: https://developers.klaviyo.com/en/reference/get_profiles
If these are all server-side calls, you can get the __kla_id cookie in the page request, decode it, get the $exchange_id, and use that value as a _kx filter to get the actual profile, and its properties. Then you can render whatever profile properties you want in the HTML you’re returning.
Get Profiles has to be called server-side since it uses a private key.
The v2 APIs are deprecated; use Get Profiles: https://developers.klaviyo.com/en/reference/get_profiles
If these are all server-side calls, you can get the __kla_id cookie in the page request, decode it, get the $exchange_id, and use that value as a _kx filter to get the actual profile, and its properties. Then you can render whatever profile properties you want in the HTML you’re returning.
Get Profiles has to be called server-side since it uses a private key.
Brilliant. By chance do you know of any example I might be able to follow to accomplish this task??
I don’t have any code snippets offhand. You’d fetch the __kla_id cookie in the request header, base64 decode it, get the $exchange_id in the JSON object it decodes to, and find the profile by using that $exchange_id in a _kx filter to Get Profiles.
I don’t have any code snippets offhand. You’d fetch the __kla_id cookie in the request header, base64 decode it, get the $exchange_id in the JSON object it decodes to, and find the profile by using that $exchange_id in a _kx filter to Get Profiles.
I feel i am so close and hoping you can solve this. I have spent over a month to figure this out
I'm trying to fetch a user profile based on the __kla_id cookie and its $exchange_id (_kx) field using the Klaviyo Get Profiles API. However, I keep getting the following error:
{
"errors": r{
"id": "555791f4-b704-458a-9900-914697adc2f0",
"status": 400,
"code": "invalid",
"title": "Invalid input.",
"detail": "Invalid _kx token",
"source": {"pointer": "_kx"},
"links": {},
"meta": {}
}]
}
Steps We've Taken:
1. Extracted the __kla_id cookie from the user's browser:
Used document.cookie.split() to find and extract the __kla_id cookie.
Base64 decoded the cookie to get a JSON object containing the $exchange_id.
2. Decoded the __kla_id:
Extracted the $exchange_id value from the decoded cookie. In this case, the _kx (exchange_id) is: T-sA-K49HXWZbSppmX1zOA.XWtsUa.
3. Used the Get Profiles API with the _kx token:
We made a cURL request to the Get Profiles API with the following command:
curl --request GET \
--url 'https://a.klaviyo.com/api/profiles/?filter=equals(_kx,"T-sA-K49HXWZbSppmX1zOA.XWtsUa")' \
--header 'Authorization: Klaviyo-API-Key PPRIVATE_KEY_HERE]' \
--header 'Accept: application/json' \
--header 'Revision: 2023-02-22'
This returned the error: "Invalid _kx token".
What We're Trying to Achieve:
Our goal is to retrieve the first name (and potentially other profile data) of the user based on the _kx (from the $exchange_id field) without using the user's email directly.
We are working on a multi-site WordPress setup and using the Klaviyo API to dynamically display profile information for users based on their __kla_id cookie.
Questions:
- Is there something we're missing in how we are decoding or passing the _kx token?
- Is the _kx token format correct, and can it always be used to fetch profiles via the Get Profiles API?
- Are there specific cases or limitations where the _kx token might not be valid?
Any guidance on why the _kx token might be rejected or how we can successfully fetch profile data based on the __kla_id
would be much appreciated!
WORKED!
FYI HOW TO DO THIS.
Step-by-Step Guide: Displaying User's First Name Based on Klaviyo's _kla_id Cookie
-
Objective: The goal was to fetch the _kla_id cookie from the user's browser, decode it to extract the _kx (exchange_id), and then use that _kx to fetch the user’s profile via the Klaviyo API and display their first name on the webpage.
-
Environment:
- WordPress site using the Divi theme
- Snippet plugin for custom PHP code
- JavaScript in Divi Theme Options for front-end processing
Steps:
1. Setting up the REST API in WordPress:
- We added a REST API endpoint in WordPress to call the Klaviyo API with the _kx (exchange ID) to retrieve the user profile.
- This was done via the Snippet plugin to handle server-side logic. Here's the PHP code for creating the custom API endpoint:
add_action('rest_api_init', function () { register_rest_route('custom-klaviyo/v1', '/get-user-profile/', array( 'methods' => 'GET', 'callback' => 'get_user_profile', )); }); function get_user_profile(WP_REST_Request $request) { $kx = $request->get_param('kx'); // Get the _kx (exchange_id) from request params if ($kx) { // Private API Key $api_key = 'YOUR_PRIVATE_API_KEY'; // Replace with your private key // Klaviyo API URL with _kx filter $url = 'https://a.klaviyo.com/api/profiles/?filter=equals(_kx,"' . $kx . '")'; // Initialize cURL session $ch = curl_init($url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_HTTPHEADER, , 'Authorization: Klaviyo-API-Key ' . $api_key, 'Accept: application/json', 'Revision: 2023-02-22', ]); // Execute the cURL request $response = curl_exec($ch); curl_close($ch); // Decode the response $profile_data = json_decode($response, true); // Extract and return the first name if available if (isset($profile_datat'data']'0]0'attributes']''first_name'])) { return n'first_name' => $profile_datat'data']'0]0'attributes']''first_name']]; } else { return n'error' => 'Profile not found or missing first name.']; } } else { return n'error' => 'No _kx provided.']; } }
- This snippet registers a new REST API endpoint at
/fstreet/wp-json/custom-klaviyo/v1/get-user-profile
. When called, it uses the _kx (exchange ID) from the _kla_id cookie to query the Klaviyo API and fetch the profile data.
2. Front-end JavaScript for Fetching the _kla_id Cookie:
-
Next, we needed to fetch the _kla_id from the user's browser, decode it to extract the _kx (exchange ID), and send it to the REST API we set up earlier.
-
This JavaScript was added to Divi Theme Options (under the Head section):
<script> document.addEventListener('DOMContentLoaded', function () { // Extract __kla_id cookie var kla_id_cookie = document.cookie.split('; ').find(row => row.startsWith('__kla_id')); if (kla_id_cookie) { var kla_id = kla_id_cookie.split('=')'1]; console.log("Klaviyo ID found: ", kla_id); // Log the __kla_id for debugging // Base64 decode the __kla_id to extract the JSON object var decoded_kla_id = atob(kla_id); var kla_id_data = JSON.parse(decoded_kla_id); // Extract the exchange_id (_kx) var exchange_id = kla_id_datat"$exchange_id"]; console.log("Exchange ID (_kx):", exchange_id); // Log the _kx // Send the exchange_id to the WordPress REST API to fetch the user profile fetch('/fstreet/wp-json/custom-klaviyo/v1/get-user-profile?kx=' + exchange_id) .then(response => response.json()) .then(profile => { if (profile.first_name) { document.getElementById('user-first-name').innerText = 'Hello, ' + profile.first_name + '!'; } else { console.log('No profile found'); document.getElementById('user-first-name').innerText = 'Profile not found.'; } }) .catch(error => console.error('Error fetching user profile:', error)); } else { console.log("No Klaviyo ID found."); document.getElementById('user-first-name').innerText = 'No Klaviyo ID found.'; } }); </script>
- The script grabs the _kla_id cookie from the browser, decodes it to extract the _kx (exchange ID), and then sends this _kx to the WordPress API endpoint to fetch the user's profile information.
3. HTML for Displaying the User's Name:
- On the Divi page, we added a placeholder for displaying the user's name once it's fetched from the API. This is simply done by placing the following HTML in the content of the page:
<div id="user-first-name"></div>
- The JavaScript will update this element with a message like "Hello, ,First Name]!" if the profile is found.
Final Results:
- Once this setup is complete, the website fetches the user's _kla_id from the browser, sends it to the custom WordPress API to retrieve the user's first name, and displays it on the webpage.
Awesome! One note: you’ll want to use the latest revision header. I don’t think anything’s changed for Get Profiles, but for new code, best to use the latest stable revision.
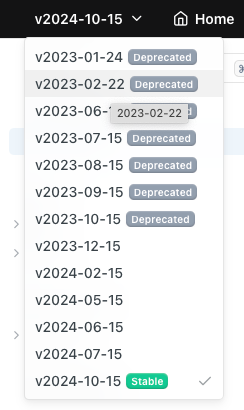
will do!
Hey,
Were you able to figure out why you got that "Invalid _kx token" error? I extracted it using the same method you did, and in most cases it works, but sometimes I get this error still.
Reply
Log in to the Community
Use your Klaviyo credentials
Log in with Klaviyo
Use your Klaviyo credentials
Log in with KlaviyoEnter your E-mail address. We'll send you an e-mail with instructions to reset your password.